

Compute and print the maximum pixel value of the clockwise rotated image. Write the clockwise rotated image to a file. Display the clockwise rotated image on the screen. (Follow similar substeps as above.) - Create an image from the rotated matrix. Create a new numpy matrix which is the original matrix rotated by 90 degrees clockwise. Write the counterclockwise rotated image to a file. Display the counterclockwise rotated image on the screen. Create an image from the rotated matrix. Use nested for loops to copy the pixel values from the original matrix image to the counterclockwise rotated one.
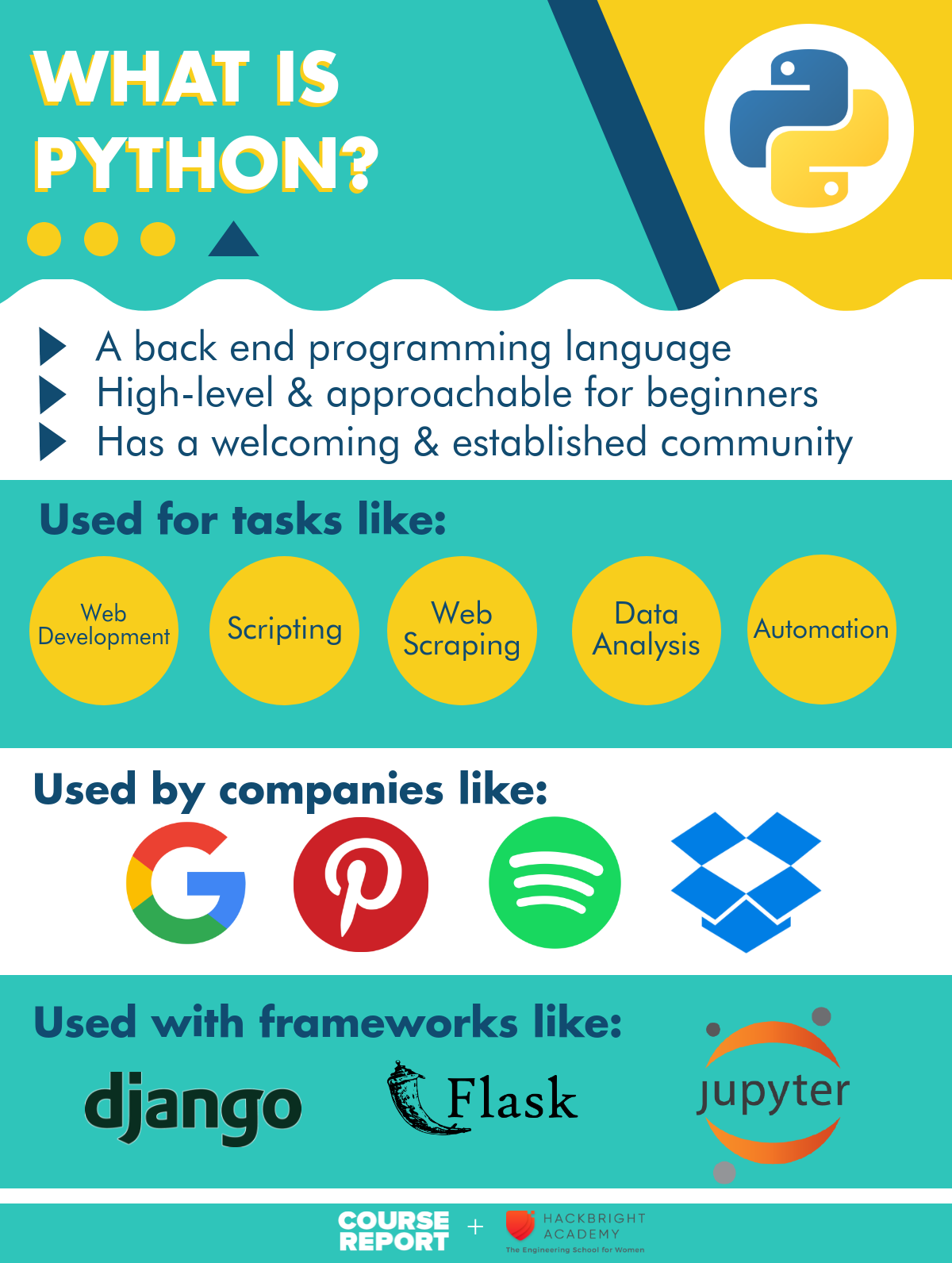
Steps to do this: - Create a new blank numpy matrix. Your new matrix should have dimensions 533 × 800 (but don't hardcode these values-use the number of rows and columns of the Beginnings grayscale image). The Beginnings grayscale image should have dimensions 800 (rows) x 533 (columns). Create a new numpy matrix which is the original matrix rotated by 90 degrees counterclockwise. Note, you cannot use any built-in functions to compute the maximum-you must loop through the pixel values. Use nested for loops to compute the maximum pixel value in the numpy matrix and print this value out to the terminal. Create a numpy matrix that has the pixel values from the image. Convert the image from color to grayscale. Create the Python file Task1.py to do the following: - Read the image "Beginnings.jpg". Task 1: Computing the maximum value of an image. This example file demonstrates a number of things that can be useful for the tasks below such as reading an image from a file, creating a grayscale image from a color one, creating a numpy matrix with the pixel values from an image, creating a blank numpy matrix and changing its values, and creating an image from a numpy matrix which contain its pixel values. See Task_example.py for an example Python file that creates a new image which is the "Beginning.jpg" image cropped by 20 pixels along each edge.
#SIMPLEIMAGE PYTHON PDF#
The goal of this lab is to make sure you are able to read and write images, convert an image from color to grayscale, perform simple operations like rotating an image 90 degrees both clockwise and counterclockwise, create a function that computes the inverse of an image, and prepare a single PDF to submit as your lab report. Title: Simple Image Manipulations in Python. Im_gray_crop.save("Beginnings_grayscale_crop.jpg") Im_gray_crop = omarray(np.uint8(im_gray_crop_pixels)) # Create an image from im_gray_crop_pixels. Im_gray_crop_pixels = im_gray_pixels[row, # Copy values from center of original matrix to smaller matrix. Im_gray_crop_pixels = np.zeros(shape=(crop_rows, crop_cols)) # Create a new matrix this is 40 pixels smaller along each Print("Image size is: ", rows, "rows x", cols, "columns") # Get access to the pixel values through the matrix Follow each steps and provide the python code.
